Functions
Methods in Go
Methods are functions which have a receiver argument (belonging to a predefined type).
Syntax
func (variableName typeName) methodName(paramName paramType) (returnVarName returnType) {
//some operation, usually on fields of the type
)
//methods are called on the variable of its type
variableName := typeName{ values for struct }
variableName.methodName(params)
Notes
Methods are typically used to emulate class-like behavior on struct types. Methods do not have to receive a struct type.
Pointers can also be receiving types for methods.
Methods behave exactly the same as functions.
Example
package main
import "fmt"
type Degrees struct {
angle float64
}
//method
func (deg Degrees) toRad() float64 {
return deg.angle * (3.14 / 180.0)
}
func main() {
angle := Degrees { 90.0 }
fmt.Println("Angle, in radians: ", angle.toRad())
}
See Also
RelatedDocumentation
The Go Programming Language Specification - The Go Programming Language
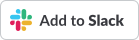