Functions
Function Literal in Go
A function literal is a function declared inline without a name.
In all other languages, this is called an anonymous function.
Syntax
func(paramName dataType) (returnVarName returnType) {
//code to execute
returnVarName = value
return value //if no return variable name specified but returnType specified
return //if return variables are assigned in the function
}
Notes
Most often used in return statements within closures.
Example
package main
import "fmt"
func square(x int) func() int {
return func() int { //anonymous function
x = x * x
return x
}
}
func main() {
twoSquared := square(2)
fmt.Println("2 squared is: ", twoSquared)
}
See Also
RelatedDocumentation
The Go Programming Language Specification - The Go Programming Language
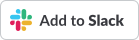