Functions
Interfaces in Go
Interfaces are types that contain several method signatures (method name declarations without implementation).
Syntax
type interfaceName interface {
methodName(params) returnType //can be several
}
//methods are implemented for several different struct types, and all methods need to be implemented
func (typeVar typeName) methodName(params) returnType {
}
//now, any type with interface method implementation can implement the interface
var interfaceVar interfaceName
typeVariable = typeName{params}
interfaceVar = typeVariable //implementing the interface for that type variable
//now, all methods in the interface can be called with respect to that type variable
Notes
There is no method implementation in an interface declaration.
Variables whose type does not implement the interface cannot be assigned to an interface variable.
There is no explicit 'implements' keyword.
Example
package main
import "fmt"
type Conversion interface {
convert() float64
}
type Degrees struct {
angle float64
}
type Radians struct {
angle float64
}
//method implementations
func (deg Degrees) convert() float64 {
return deg.angle * (3.14 / 180.0)
}
func (rad Radians) convert() float64 {
return rad.angle * (180.0 / 3.14)
}
func main() {
var c Conversion
r := Radians{1.57}
c = r //implementing the conversion interface
fmt.Println("1.57rad, in degrees: ", c.convert())
var cDeg Conversion = &Degrees{90}
fmt.Println("90 degrees, in radians: ", cDeg.convert())
}
See Also
RelatedDocumentation
The Go Programming Language Specification - The Go Programming Language
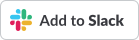