Object Oriented Programming
Throwing an Exception in Java
Methods prone to an error in runtime can throw an exception, instead of having program crash or having a logical error within the program.
Syntax
public methodName function throws exceptionName{
//checking for regular or error condition
if(booleanExpression){ //good condition
}
else { //error condition
throw new exceptionName();
}
}
Notes
Exceptions are typically thrown when a method itself doesn't handle a checked exception.
Example
public createArray() throws ArrayIndexOutOfBoundsException{
int[] array = new int[3];
int index = 7;
if(index < array.length){
array[index] = 3;
}
else {
throw new ArrayIndexOutOfBoundsException();
}
}
See Also
RelatedDocumentation
How to Throw Exceptions - Java Tutorials
Specifying the Exceptions Thrown by the Method
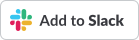