Object Oriented Programming
Declaring an Exception in Java
Apart from the built in exceptions Java provides, exception classes can be written by the programmer. These can then be thrown like the built-in Java exceptions.
Syntax
modifier exceptionName extends Exception {
//fields and methods
}
modifier exceptionName extends RuntimeException { //for runtime exceptions
//fields and methods
}
Notes
If the exception thrown is handled or declared, the class declared must extend the Exception class.
If the exception thrown is a runtime exception, the class declared must extend the RuntimeException class.
Example
//exception for negative numbers
public negativeNumberException extends Exception { //class header
private int errorNumber;
public negativeNumberException(int number){ //exception class constructor
this.errorNumber = number;
}
public int getNumber() { //method within class
return this.errorNumber;
}
}
//a class that only takes positive numbers
public class positiveNumbers {
private int number;
//method within class throws negative number exception
public positiveNumber(int number) throws negativeNumberException{
if(number >= 0) this.number = number;
else {
throw new negativeNumberException(number);
}
}
}
//trying the exception, elsewhere in the code
try {
positiveNumbers negativeOne = new positiveNumbers(-1);
} catch (negativeNumberException e) {
System.out.println("ERROR: This number is not positive: " + e.getNumber());
}
See Also
RelatedTry Catch Block
Throwing an Exception
Creating Exception Classes - Java Tutorials
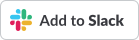