Variables
Variable Declaration in C#
Used to declare a variable. Variables can be implicitly or explicitly typed.
Variables declared this way (without a static modifier) within classes are called instance variables. They belong to an instance of the class (i.e. an object).
Syntax
modifier dataType variableName; ///modifier is optional
///variables can be assigned values either separately or on declaration
variableName = value; ///separate line assignment
modifier dataType variableName = value; ///same line assignment
///implicitly typed variable
var variable2 = value;
Notes
The modifier (public, private) permits or restricts direct access to the variable with respect to its scope (class, method, etc.). Variables declared within a class are called fields.
Variables without a modifier are known as local variables, typically used within a method. They are temporary and only exist within the scope of the where its declared method.
dataType is the data type of the variable.
Example
public class Car {
private int speed; ///private variable declaration
public int wheels; ///public variable declaration
/*...constructor, etc...*/
public void speedUp() {
///local variable declaration, in line assignment, only seen within speedUp method
int speedIncrease = 10;
speed += speedIncrease;
}
}
See Also
RelatedAccess Modifiers
Primitive Data Types
Types (C# Programming Reference) - MSDN
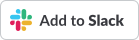