Functions
Functions in Swift
A function is a block of code that can be called from another location in the program or class.
Functions declared within a class are known as methods. Specifically, they are declared as below, they are known as instance methods.
Syntax
func functionName(paramName: dataType, param2: dataType) -> returnDataType {
//code to execute
return value //optional return statement
}
//passed parameters can be associative (optional)
functionName(param1Value, passedParam2: param2Value)
Notes
If you want to force parameters to be passed associatively, use '#' before your parameter in your function declaration. This is known as an external parameter.
Values passed into the function are stored inside the parameter variables. These parameter variables have local function scope, which means if they are modified, changes will not be seen outside of the function. To make these changes permanent, use the 'inout' keyword before the parameter name.
Multiple values can be returned in a function. After the ->, use brackets and specify the return names and types (returnVar1: returnDataType1, returnVar2: returnDataType2). In the return statement, return the values in brackets (returnValue1, returnValue2).
Optionals can be used as return data types.
In Swift, a function with a return type does not have to be assigned to anything when called.
Example
func findMax(a: Double, b: Double) -> Double {
return a > b ? a : b
}
let max = findMax(3, 5)
See Also
RelatedDocumentation
The Swift Programming Language - Functions
The Swift Programming Language - Methods
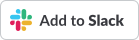